Client Side AJAX Applications in SharePoint 2010 – Part 5: Modal Dialogs
Guest Author: Lee Richardson
http://rapidapplicationdevelopment.blogspot.com/
A significant portion of SharePoint 2010’s Web 2.0 look and feel derives from its use of modal popups. This post deviates briefly from the ASP.Net AJAX Templating discussion to explore how to open remote pages as modal dialogs, how to open dialogs based on local HTML, and how to respond to OK or Cancel events.
Modaling to Another Page
To get started let’s throw an edit icon onto the index cards we’ve built previously that will open a modal dialog:
<div class="userStoryTitle"> {{ Title }} <span class="userStoryButtons" sys:command="select"> <a href="#" onclick="javascript:openDialog(); return false;"> <img src="/_layouts/images/edititem.gif" /> </a> </span> </div>
There’s nothing fancy going on here yet, just a call to an as yet unimplemented openDialog() function. The result looks like this:
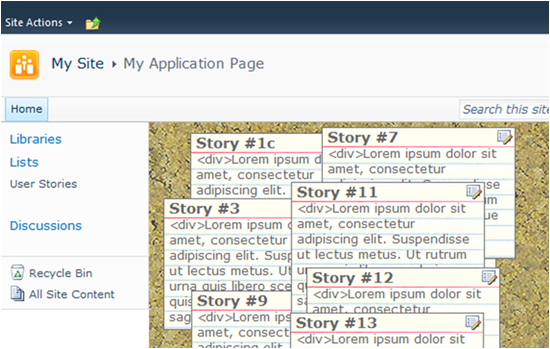
To open an AJAX modal dialog in SharePoint 2010 either specify a remote page to open, or specify an HTML element in the page. Opening a remote page is a little easier. The openDialog() function would look like this:
function openDialog() { var options = { url: "../../Lists/User%20Stories/DispForm.aspx?Id=1", width: 800, height: 600, title: "User Story", }; SP.UI.ModalDialog.showModalDialog(options); }
Obviously hard coding an id in the url parameter isn’t exactly best practice, but it works for demonstration purposes. The result looks like this:
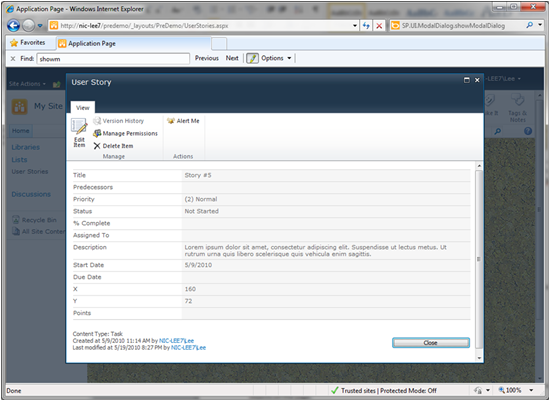
This is a useful technique for opening existing SharePoint pages as in the example above or for opening pages as a dialog that a user might also navigate to directly. However, in our application we want to edit information that is in-memory in the browser (which is where ASP.Net AJAX Templating stores its data). The showModalDialog() function will support this scenario, but it’s slightly more complicated.
Modaling to Your Own Page
First we’ll need an HTML element that we’re going to pop up. A first draft might looks like this:
<div id="userStoryDetails"> Hello World! </div>
Since the options parameter that we passed to showModalDialog() supports an ‘html’ parameter instead of a ‘url’ parameter it might seem like we could simply retrieve the userStoryDetails element inside of openDialog and pass that to options. However, there’s a problem with this approach. By default SharePoint’s showModalDialog() function will destroy the DOM element that are passed to it. The result is that the dialog can be opened once, but it fails each subsequent time it is opened.
To avoid this behavior we can cache the DOM element in a global variable rather than keeping it as a local variable scoped at the function level. The code to will look like this:
var userStoryDetails; Sys.onReady(function () { userStoryDetails = document.getElementById("userStoryDetails"); ... }); function openDialog() { var options = { html: userStoryDetails, width: 600, height: 300, title: "User Story", }; SP.UI.ModalDialog.showModalDialog(options); }
With this code we can close and open the modal dialog multiple times. The result looks like this:
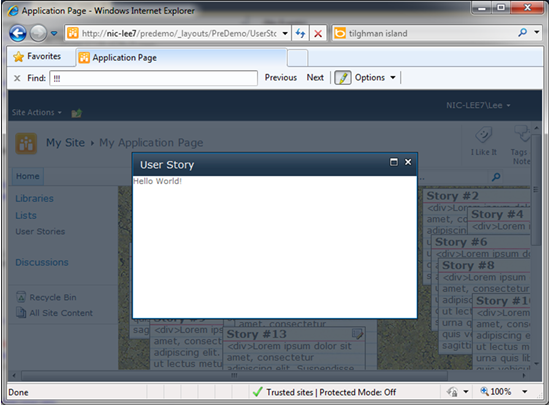
The only thing we’re missing now is the ability to close the dialog and handle the results.
Goodbye World
Closing the dialog and handling results is also pretty simple. It takes two steps. First we’ll need to call commonModalDialogClose() in our popup code and next we’ll implement a callback method that SharePoint will call when the dialog has been closed.
To call commonModalDialogClose() we can modify the code that we are going to pop up like this:
<div id="userStoryDetails"> <input type="button" value="OK" onclick="SP.UI.ModalDialog.commonModalDialogClose(SP.UI.DialogResult.OK, 'Ok clicked'); return false;" class="ms-ButtonHeightWidth" target="_self" /> <input type="button" value="Cancel" onclick="SP.UI.ModalDialog.commonModalDialogClose(SP.UI.DialogResult.cancel, 'Cancel clicked'); return false;" class="ms-ButtonHeightWidth" target="_self" /> </div>
Notice the first parmeter to commonModalDialogClose(). It’s a DialogResult. This parameter is important because our callback method will need to differentiate the ways in which a user could close the dialog. For instance when a user clicks the X in the upper right of the dialog SharePoint passes DialogResult.cancel for the first parameter.
The second parameter to commonModalDialogClose() is simply passed through as the second parameter to our handler as we’ll see shortly.
To handle the results of closing the dialog we can pass a function to the dialogReturnValueCallback argument in the options parameter of showModalDialog. The result would look like this:
function openDialog() { var options = { html: userStoryDetails, width: 800, height: 600, title: "User Story", dialogReturnValueCallback: onDialogClose }; SP.UI.ModalDialog.showModalDialog(options); } function onDialogClose(dialogResult, returnValue) { if (dialogResult == SP.UI.DialogResult.OK) { alert('goodbye world!'); } if (dialogResult == SP.UI.DialogResult.cancel) { alert(returnValue); } }
And now we can open and close SharePoint modal dialogs and handle the results. If needed there are other adjustments we can make to modal dialogs through the options parameter. For instance X, Y, allowMaximize, showMaximized and showClose. The documentation is sparse, but hopefully this page on MSDN will eventually be updated with more details.
Summary
This post should provide everything needed for opening dialogs that look like the ones SharePoint uses. And more importantly it will provide a foundation for the next post in the series in which we will edit user story information using a master-details view inside of a modal popup.
Guest Author: Lee Richardson
http://rapidapplicationdevelopment.blogspot.com/
Lee Richardson is a Senior Software Engineer at Near Infinity Corporation, an enterprise software development and consulting services company based in Reston, Virginia. He is a Microsoft Certified Solution Developer (MCSD), a Project Management Professional (PMP), a Certified SCRUM master and has over ten years of experience consulting for the public and private sector. You can follow Lee on Twitter at @lprichar
- Client Side AJAX Applications in SharePoint 2010 – Part 1: Introduction
- Client Side AJAX Applications in SharePoint 2010 – Part 2: WCF Data Services
- Client Side AJAX Applications in SharePoint 2010 – Part 3: ASP.Net AJAX Templating 101
- Client Side AJAX Applications in SharePoint 2010 – Part 4: jQuery Integration and Persistence
- Client Side AJAX Applications in SharePoint 2010 – Part 5: Modal Dialogs
- Client Side AJAX Applications in SharePoint 2010 – Part 6: Master-Details
- Client Side AJAX Applications in SharePoint 2010 – Part 7: Live Bindings
hello, is there a way to add a dialog to open a newform?
basically, I’m looking to add a left menu link or on page link to open a dialog box to create a new item. I’m hoping to do this with javascript.
I created a SP List with custom Content Type. When I create new item or Edit a item, a pop-up will show, How can I custom this pop-up as I want.
Thank you.
Hi Lee,
I just want the ability to pop up images in an Ajax dialog. Is there an easy way to do this?
Thanks
Dave
HI!!
I have an web page in sharepoint 2010 with a link. I want to open a modal Dialog bu each time I save the change in SharePoint designer is remove the lines of the href=”javascript:function();”. How can I call a javascript funcion and what reference should I have to be enable to open a web page in the modal dialog??
Thanks!
Hank
Excellent article. I want to pass dynamic id as the list item changes – any idea
Ex: url: “../../Lists/User%20Stories/DispForm.aspx?Id=”+ID,
@Abhi:
You can use the following for dynamic list entries:
~site – URL relative to the current web site (SPWeb)
~sitecollection – URL relative to the current site collection (SPSite)
{ItemId} – ID that identifies the item within a list
{ItemUrl} – URL of the current item
{ListId} – GUID of the current list
{SiteUrl} – Absolute URL of the current web site (SPWeb)
I highly recommend the following book for development on SharePoint 2010: SharePoint 2010 as a Development Platform by Jorg Krause, Christian Langhirt, Alexander Sterff, Bernd Pehlke, Martin Doring. It is published by Apress. ISBN: 978-1-4302-2706-9
Hi, Thanks a lot for your article. It helped me built the popups for our application.
But I’m having a problem with calendarextender on the modaldialog.
I’m using the modaldialog with a div, in that div I have a textbox with calendarextender and it raises a javascript error wich says that 2 objects with same id can’t be added.
I changed to a regular asp calendar and also gives an error. This one I undestand that is sayng that the event was not fired by the correct control.
Also, in wss 3.0 I had html/javascript popups. I created 2 divs (background and front) with position absolute and it worked. In sharepoint 2010 the position:absolute does not work. It’s always relative to the webpart content holder. That’s why I’m changing to modaldialogs.
Can you help?
Thanks in advance
Bruno Henriques
Hi, i managed to solve my problems from the previous comment.
But there’s still a problem.
I have a div wich is showed in the dialog. It has 2 buttons, ok and cancel and when the user clicks on OK i must execute a function in codebehind, but he button does not generate a postback.
How can I execute this?
Thanks in advance
Bruno Henriques
The code works great for adding a link on my site that launches the upload an image dialog window.
I now need to do the same thing in a 2007 MOSS site. Anyone know how to that?
Hi, im wondering if your solution can be used to fulfill my client requirement. I need some way of displaying a pop up for all users when a new announcement is added to the announcements list so that they are made aware on screen of the precense opf the announcement. They are not satisfied with alerts to outlook. the contwent of the pop up will be the headline of the announcement, maybe along with the url to the item? Thanks in advance