JQuery for Everyone: Fixing Configured Web Part Height
Problem: When you set the height of a web part, it will still grow beyond that height to fit the height of the contents.
Solution: Use jQuery to change CSS values and element attributes to correct this for any web part with a configured height.
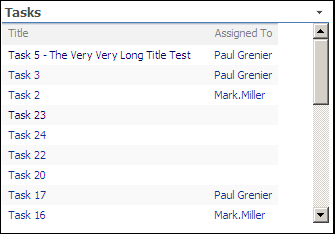
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.2.6/jquery.min.js" type="text/javascript"></script> <script type="text/javascript"> $(function() { //creates scrollbar for web part with configured height var arrayList = $("div[id^='WebPartWPQ']").get(); $.each(arrayList, function(i,e){ var str = $(e).attr("style").toString().toUpperCase(); if (str.indexOf("HEIGHT") >= 0) { //need caps for diff browsers $(e).css({"overflow-x":"hidden","overflow-y":"auto"}) .find("table.ms-summarystandardbody").removeAttr("width"); } }); }); </script>
Author: Paul Grenier
- JQuery for Everyone: Accordion Left Nav
- JQuery for Everyone: Print (Any) Web Part
- JQuery for Everyone: HTML Calculated Column
- JQuery for Everyone: Dressing-up Links Pt1
- JQuery for Everyone: Dressing-up Links Pt2
- JQuery for Everyone: Dressing-up Links Pt3
- JQuery for Everyone: Cleaning Windows Pt1
- JQuery for Everyone: Cleaning Windows Pt2
- JQuery for Everyone: Fixing the Gantt View
- JQuery for Everyone: Dynamically Sizing Excel Web Parts
- JQuery for Everyone: Manually Resizing Web Parts
- JQuery for Everyone: Total Calculated Columns
- JQuery for Everyone: Total of Time Differences
- JQuery for Everyone: Fixing Configured Web Part Height
- JQuery for Everyone: Expand/Collapse All Groups
- JQuery for Everyone: Preview Pane for Multiple Lists
- JQuery for Everyone: Preview Pane for Calendar View
- JQuery for Everyone: Degrading Dynamic Script Loader
- JQuery for Everyone: Force Checkout
- JQuery for Everyone: Replacing [Today]
- JQuery for Everyone: Whether They Want It Or Not
- JQuery for Everyone: Linking the Attachment Icon
- JQuery for Everyone: Aspect-Oriented Programming with jQuery
- JQuery for Everyone: AOP in Action - loadTip Gone Wild
- JQuery for Everyone: Wiki Outbound Links
- JQuery for Everyone: Collapse Text in List View
- JQuery for Everyone: AOP in Action - Clone List Header
- JQuery for Everyone: $.grep and calcHTML Revisited
- JQuery for Everyone: Evolution of the Preview
- JQuery for Everyone: Create a Client-Side Object Model
- JQuery for Everyone: Print (Any) Web Part(s) Plugin
- JQuery for Everyone: Minimal AOP and Elegant Modularity
- JQuery for Everyone: Cookies and Plugins
- JQuery for Everyone: Live Events vs. AOP
- JQuery for Everyone: Live Preview Pane
- JQuery for Everyone: Pre-populate Form Fields
- JQuery for Everyone: Get XML List Data with OWSSVR.DLL (RPC)
- Use Firebug in IE
- JQuery for Everyone: Extending OWS API for Calculated Columns
- JQuery for Everyone: Accordion Left-nav with Cookies Speed Test
- JQuery for Everyone: Email a List of People with OWS
- JQuery for Everyone: Faster than Document.Ready
- jQuery for Everyone: Collapse or Prepopulate Form Fields
- jQuery for Everyone: Hourly Summary Web Part
- jQuery for Everyone: "Read More..." On a Blog Site
- jQuery for Everyone: Slick Speed Test
- jQuery for Everyone: The SharePoint Game Changer
- JQuery For Everyone: Live LoadTip
Paul
This might seem like a silly question, but is it safe to reference the library at:
http://ajax.googleapis.com/ajax/libs/jquery/1.2.6/jquery.min.js
Or should I reference it locally in our Farm?
Regards
Paul
If your site uses SSL, you will want to download the minified .js file and store it locally (server-level, library-level, or using a manager like ShUIE).
And always for best performance, store it locally.
Otherwise, if you can’t trust Google, who can you trust? Seriously, there are benefits to using Google: (1) the more sites use it, the more likely caching the file will speed performance; (2) Google will update the code for you; (3) your entire code snippet is as easy as “drag-n-drop”.
That’s my main reason for using it here. No need to modify that snippet to test it out locally (unless your firewall blocks Google-pshaw!).
awesome! thanks a lot.
If you would like to add the jQuery library to your local SharePoint server, instead of downloading it from Google, you can use the SmartTools.jQuery feature. More info: http://weblogs.asp.net/jan/archive/2008/11/20/sharepoint-2007-and-jquery-1.aspx or get it on CodePlex: http://www.codeplex.com/smarttools/
What benefit is there to using jquery to set the css vs just setting it with an overide css file. Particularly if you have any type of custom branding to your site, as you would/should already have this in place. The overflow property should only come into affect if the height value is exceeded, so there’s really no use for the conditional statement. Also, setting seperate overflow x & y values isn’t supported by all browsers, so setting more browser specific css values would be a bit easier with a seperate css file (imho, makes inheritance/specificity easier to maintain). I love using jquery in my sharepoint sites, but i’m not sure what the benefit is here…
Ernie – What happens when you don’t have access to the server to put in an overide css file? The solution here gives site managers, with no server access, the ability to manage the layout of specific pages.
Granted, the solution isn’t optimal because, as you imply, it needs to be rolled out across the entire site.
Be that as it may, Paul’s solution can be used by thousands of site managers who are trying to manage specific pages on their sites.
Regards,
Mark
I’ll be the first to admit I’m no expert with this stuff and these are simple examples–not Enterprise solutions–but I can try to explain a few details:
1) Why the condition? The condition is so we only change web parts with a height configured in the web part settings pane. The default settings and behavior are fine for most web parts, so I don’t want to make global CSS changes.
2) Overflow-x/y? I tested with FF3, Chrome, and IE7. If a particular browser (like mobile) requires additional CSS, it’s fairly easy to change this script.
3) CSS vs. js? I agree, CSS is more efficient when available. In this case I could not remove the hard-coded width attribute from the table with CSS–I just wanted it gone. But again, only from web parts with a pre-configured HEIGHT.
Handy little piece of code, solved my problems straight out of the box! :)
Thanks!
Paul,
I’ve run into an issue with this. It only works for admins on the sites that I put it onto. I have jquery in the /_layouts folder on the sharepoint server and I’m thinking it might be a permissions issue for normal users accessing the jquery script.
Can you shed any light on this?
@erugalatha,
If the page is in a publishing site, make sure the page is published.
If you placed the jquery library in a subfolder of _layouts, you may need to update IIS virtual directory with the correct permissions.
Hi Paul,
Love your series more and more as I try our tricks one after the other.
Could the scrolling only start below the toobar.
I combined this feature with the expand/ collapse all and it would be better to freeze the toolbar and scroll below it.
Greg
Greg,
Looks like you could do it with the toolbar, but it would not be easy with the column headers. You basically need to have a contained to apply the new CSS to, and the column headers and data are in the same container (they’re siblings).
You will get the same result with:
Nr 3 is an example, and relates to proper WebPart ordinal.
Greg
Hello,
can you please post the code again!
I cannot find it…
Thanks
Michael
Michael – Done — Mark
One beginners question….
Wheres the css to be placed?
Like I have a page viewer webpart with the same issue. Do i put the script in the page being displayed in the webpart or somewhere else?
Mandar,
Almost always, JavaScript or CSS solutions are added to a Content Editor Webpart on the page.
If I scroll the List Item then Header Section also goes up.
What’s the way to set the Header Section as fix while scrolling the list item in above post.